Unlocking Growth with Animated Bubble Charts in JavaScript
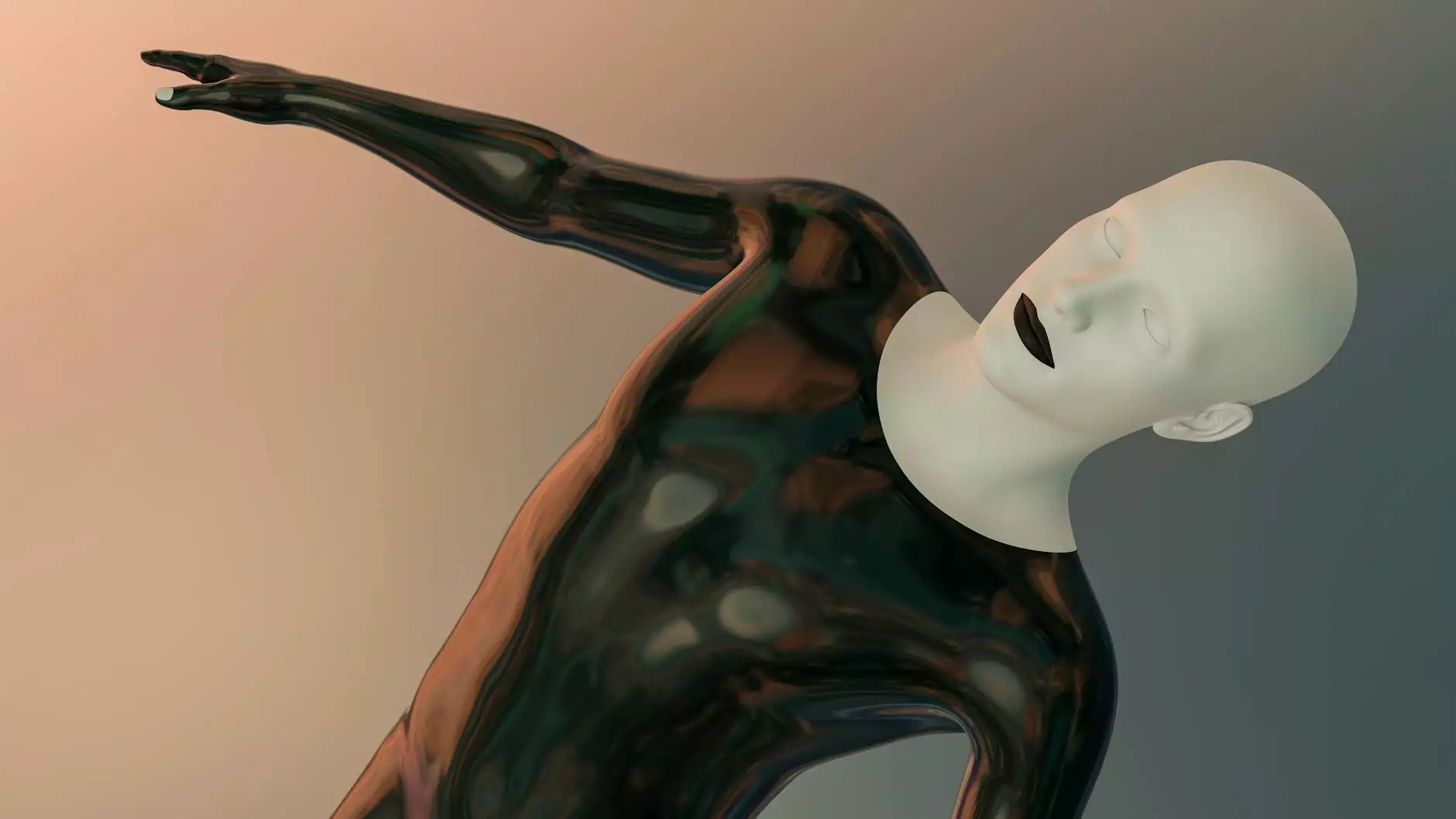
In today's data-driven business landscape, visualizing information effectively is more crucial than ever. Companies must analyze vast amounts of data to make informed decisions and implement strategies that drive growth. One powerful tool that has gained popularity in this context is the animated bubble chart in JavaScript.
What is an Animated Bubble Chart?
A bubble chart is a type of data visualization that represents three dimensions of data. The x-axis and y-axis represent two variables, while the size (and sometimes color) of the bubbles represents a third variable. When this chart is animated, it can depict changes over time, providing a dynamic view of data trends.
The Structure of an Animated Bubble Chart
- Bubbles: Each bubble corresponds to a data point.
- Axes: X and Y axes represent different metrics or variables.
- Animation: The transition effects that occur as data is manipulated over time.
Benefits of Using Animated Bubble Charts in Business
Incorporating animated bubble charts into your business strategy can yield numerous advantages:
1. Enhanced Data Visualization
Data can be difficult to comprehend in raw numeric form. Animated bubble charts simplify complex data sets, making it easier for stakeholders to visualize trends, correlations, and patterns. Moving visuals capture attention and can convey information more effectively than static graphs.
2. Improved Decision-Making
With a clearer understanding of their data, business leaders can make quicker and more informed decisions. By analyzing changes over time through animation, managers can identify opportunities and threats before they become apparent through traditional analysis.
3. Effective Communication
Visual tools bridge communication gaps among professionals from different backgrounds. An animated bubble chart can be shared in presentations, reports, and meetings, enabling teams to discuss findings and strategies without ambiguity.
4. Engaging Stakeholders
Dynamic animations stimulate engagement. Stakeholders are more likely to pay attention to animated content than static presentations. This increased engagement can lead to productive discussions and greater buy-in for strategic initiatives.
Implementing Animated Bubble Charts in JavaScript
Creating an animated bubble chart in JavaScript requires proficiency in programming and familiarity with several libraries that facilitate this process. Let's dive into a step-by-step guide.
Step 1: Choosing the Right JavaScript Library
Several libraries can help you create animated bubble charts effectively, including:
- D3.js: A powerful tool for manipulating documents based on data, great for complex visualizations.
- Chart.js: A user-friendly library that offers simple chart creation options.
- Highcharts: Known for its beautiful visuals and ease of use, ideal for interactive charts.
Step 2: Preparing Your Data
Your data should be structured correctly before you begin coding. Typically, data for a bubble chart is organized as follows:
- Label: Describes the data point.
- X Value: Represents a numeric variable displayed on the x-axis.
- Y Value: Represents a numeric variable displayed on the y-axis.
- Size: Indicates the importance of the data point, displayed through the bubble's size.
Step 3: Coding Your Chart
Here’s an example of how you can create a simple animated bubble chart using D3.js:
const data = [ { label: 'A', x: 30, y: 30, size: 60 }, { label: 'B', x: 80, y: 80, size: 30 }, // additional data points... ]; // Set dimensions and create SVG const width = 600; const height = 400; const svg = d3.select('body').append('svg') .attr('width', width) .attr('height', height); // Create bubbles const bubbles = svg.selectAll('.bubble') .data(data) .enter().append('circle') .attr('class', 'bubble') .attr('cx', d => d.x) .attr('cy', d => d.y) .attr('r', d => d.size / 2) .style('fill', 'blue') .attr('opacity', 0.7) .transition() .duration(2000) .attr('r', d => d.size);Step 4: Adding Animation
To enhance your animated bubble chart, you can add animations that trigger when data changes:
// Animate on data change function updateData(newData) { // Bind new data const bubbles = svg.selectAll('.bubble') .data(newData); // Transition existing bubbles bubbles.transition() .duration(2000) .attr('cx', d => d.x) .attr('cy', d => d.y) .attr('r', d => d.size / 2); // Enter new data points bubbles.enter().append('circle') .attr('class', 'bubble') .attr('cx', d => d.x) .attr('cy', d => d.y) .attr('r', 0) // start with no size .style('fill', 'red') .transition() .duration(2000) .attr('r', d => d.size); }Real-World Applications of Animated Bubble Charts
Understanding how to implement a bubble chart is useful, but knowing when to use it is vital. Below are some real-world applications:
1. Marketing Analytics
Marketing teams can visualize customer segmentation by plotting potential customers based on demographics (x-axis), purchasing behavior (y-axis), and purchase volume (size of bubbles). This allows for targeted marketing strategies and resource allocation.
2. Financial Analysis
Financial analysts can represent different investments where the x-axis shows risk, the y-axis shows return, and the size of the bubble indicates total investment size. This dynamic visualization helps in portfolio selection.
3. Performance Tracking
Companies can utilize bubble charts to track the performance of various business units by measuring profit margins (x-axis), growth rates (y-axis), and revenue (bubble size). This assists management in identifying high-performing sectors and areas needing attention.
Conclusion
In summary, animated bubble charts in JavaScript are an invaluable tool for modern businesses. They enhance data visualization, improve decision-making, and facilitate better communication among stakeholders. By using the right JavaScript libraries and following proper implementation techniques, companies can unlock the full potential of their data.
As businesses like Kyubit.com continue to innovate in marketing and consulting, integrating advanced visualization techniques like animated bubble charts can set them apart in the competitive landscape. Embrace this tool today, and watch your business thrive!
animated bubble chart javascript